Announcing Hazelcast Cloud Go SDK
We love Golang since it helps us to automate our SaaS business with a low resource cost. If we have a Golang project, we easily compile it into any kind of distribution as you can see here. Today, we are happy to announce the Hazelcast Cloud Golang SDK to automate your Hazelcast Cloud workloads and related operations by using Golang. If you don’t have a Hazelcast Cloud account, you can sign-up for a free one here, and get API Credentials from the Developer page.
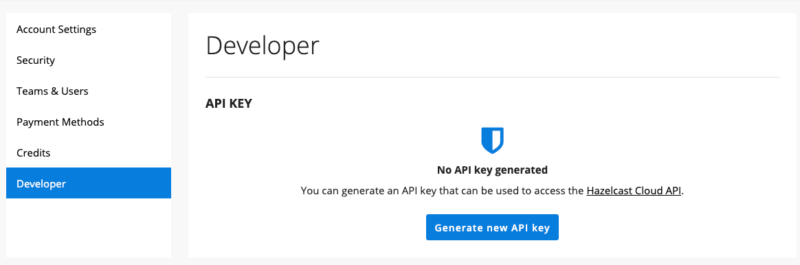
Getting Started
To start using Hazelcast Cloud Go SDK, you can just add github.com/hazelcast/hazelcast-cloud-sdk-go to your project by using a dependency management tool. In order to call Hazelcast Endpoints by using Go SDK, you need to define Hazelcast Cloud specific environment variables.
Client Configuration
To access Hazelcast Cloud Endpoints, you need to define Api Key and Api Secret within your environment variables or pass them as constructor parameters to the Golang client. Before doing that, you need to go to the Developer page, then generate your credentials.
export HZ_CLOUD_API_KEY=<API_key> export HZ_CLOUD_API_SECRET=<api_secret>
then within your golang project;
package main import ( "github.com/hazelcast/hazelcast-cloud-sdk-go" ) func main() { client := hazelcastcloud.New() }
as an alternative, you can pass credentials to your constructor;
package main import ( "github.com/hazelcast/hazelcast-cloud-sdk-go" ) func main() { client := hazelcastcloud.NewFromCredentials("<api_key>", "<api_secret>") }
Hands-on Examples
Create Cluster
clusterName := "my-awesome-cluster" createInput := models.CreateStarterClusterInput{ Name: clusterName, CloudProvider: "was", Region: "us-west-2", ClusterType: "FREE", HazelcastVersion: "4.0", TotalMemory: 0.2, } newCluster, _, createErr := client.StarterCluster.Create(context.Background(), &createInput) if createErr != nil { fmt.Printf("An error occurred: %s\n\n", createErr) return }
You can always refer to Hazelcast Cloud GoDoc for the internals, and you can see at least one example for each function exposed inside Hazelcast Cloud Golang SDK.